Django serve static files
Static files
Serving static files in Django is an essential part of web development, especially for managing assets like CSS, JavaScript, and images. Here’s a step-by-step guide to help you configure and serve static files in a Django project:
Settings Configuration
First, you need to set up your settings.py file:
-
STATIC_URL
: URL to use when referring to static files located inSTATIC_ROOT
. -
STATICFILES_DIRS
: A list of directories where Django will also look for static files, apart from each app’s static directory. -
STATIC_ROOT
: The absolute path to the directory where collectstatic will collect static files for deployment.
import os # URL to use when referring to static files (where they will be served from) STATIC_URL = 'static/' if DEBUG: STATICFILES_DIRS = [ os.path.join(BASE_DIR, 'static') # development environment ] else: STATIC_ROOT = os.path.join(BASE_DIR, 'staticfiles') # production
Collect Static Files
During development, you can serve static files directly from their locations. However, for production, you should collect all static files into the directory specified by STATIC_ROOT
using the collectstatic
command:
python3 manage.py collectstatic
This command will gather all static files from your apps and the directories listed in STATICFILES_DIRS
and place them in STATIC_ROOT
.
Serve Static Files in Development
Django can serve static files during development using the django.contrib.staticfiles
app. Ensure it’s included in your INSTALLED_APPS
:
INSTALLED_APPS = [ ... 'django.contrib.staticfiles', ... ]
Django automatically serves static files when DEBUG = True
. Just make sure your URLs are configured to handle static files:
from django.conf import settings from django.conf.urls.static import static from django.urls import path urlpatterns = [ ... ] if settings.DEBUG: urlpatterns += static(settings.STATIC_URL, document_root=settings.STATIC_ROOT)
Serving Static Files in Production
For production, it’s recommended to serve static files through a web server like Nginx or Apache rather than Django. Here’s an example configuration for Nginx:
server { listen 80; server_name your_domain.com; location /static/ { alias /path/to/your/project/staticfiles/; } location / { proxy_pass http://127.0.0.1:8000; # Assuming Django runs on port 8000 proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; proxy_set_header X-Forwarded-Proto $scheme; } }
Replace /path/to/your/project/staticfiles/
with the actual path to your STATIC_ROOT
.
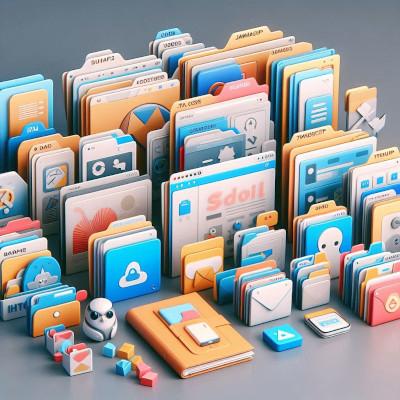
Testing Your Configuration
After setting everything up, you can test your configuration:
1- Run your Django development server:
python3 manage.py runserver
2- Navigate to one of your static files in the browser, for example:
http://127.0.0.1:8000/static/yourfile.css
In production, after configuring your web server, restart the server and access a static file via your domain to ensure everything is set up correctly.